Advanced Python
In this instructor-led, live training, participants will learn advanced Python programming techniques, including how to apply this versatile language to solve problems in areas such as distributed applications, data analysis and visualization, UI programming and maintenance scripting.
Format of the Course
- Interactive lecture and discussion.
- Lots of exercises and practice.
- Hands-on implementation in a live-lab environment.
Course Customization Options
- If you wish to add, remove or customize any section or topic within this course, please contact us to arrange.
Requirements
- Beginner to intermediate programming experience.
- Knowledge of math and statistics.
- Knowledge of database concepts.
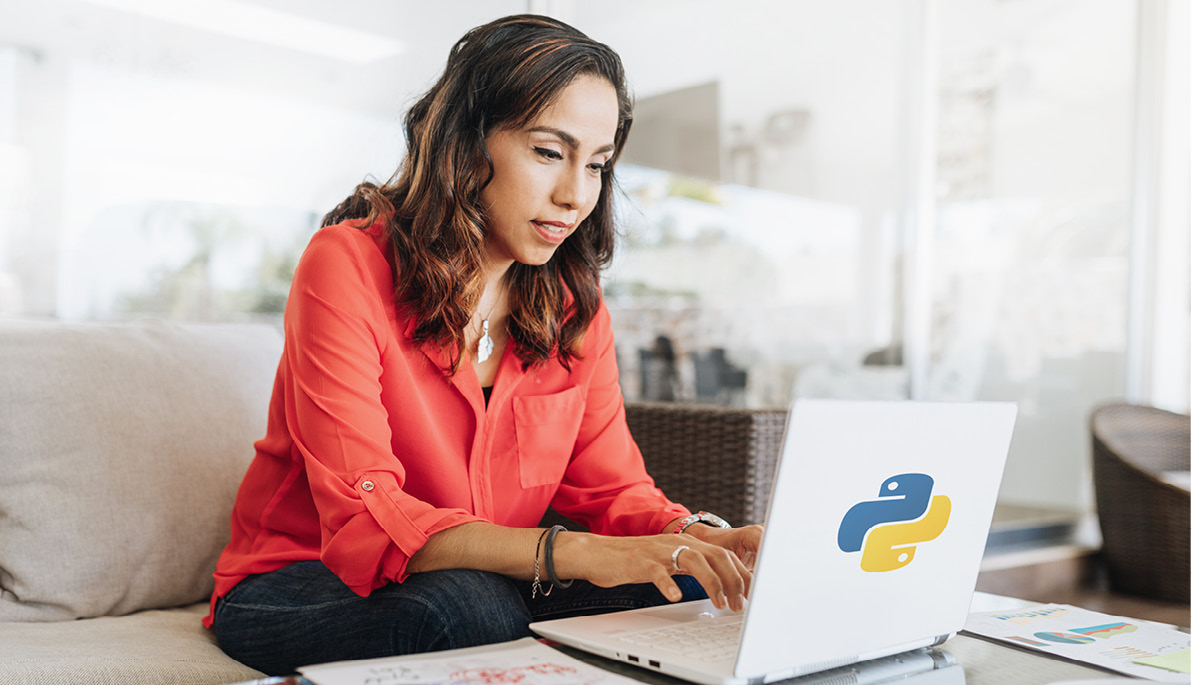
Course Outlines
Introduction
- Python versatility: from data analysis to web crawling
Python Data Structures and Operations
- Integers and floats
- Strings and bytes
- Tuples and lists
- Dictionaries and ordered dictionaries
- Sets and frozen sets
- Data frame (pandas)
- Conversions
Object-Oriented Programming with Python
- Inheritance
- Polymorphism
- Static classes
- Static functions
- Decorators
- Other
Data Analysis with Pandas
- Data cleaning
- Using vectorized data in pandas
- Data wrangling
- Sorting and filtering data
- Aggregate operations
- Analyzing time series
Data Visualization
- Plotting diagrams with matplotlib
- Using matplotlib from within pandas
- Creating quality diagrams
- Visualizing data in Jupyter notebooks
- Other visualization libraries in Python
Vectorizing Data in Numpy
- Creating Numpy arrays
- Common operations on matrices
- Using ufuncs
- Views and broadcasting on Numpy arrays
- Optimizing performance by avoiding loops
- Optimizing performance with cProfile
Processing Big Data with Python
- Building and supporting distributed applications with Python
- Data storage: Working with SQL and NoSQL databases
- Distributed processing with Hadoop and Spark
- Scaling your applications
Extending Python (and vice versa) with Other Languages
- C#
- Java
- C++
- Perl
- Others
Data Serialization
- Python object serialization with Pickle
Python Multi-Threaded Programming
- Modules
- Synchronizing
- Prioritizing
UI Programming with Python
- Framework options for building GUIs in Python
- Tkinter
- Pyqt
Python for Maintenance Scripting
- Raising and catching exceptions correctly
- Organizing code into modules and packages
- Understanding symbol tables and accessing them in code
- Picking a testing framework and applying TDD in Python
Python for the Web
- Packages for web processing
- Web crawling
- Parsing HTML and XML
- Filling web forms automatically